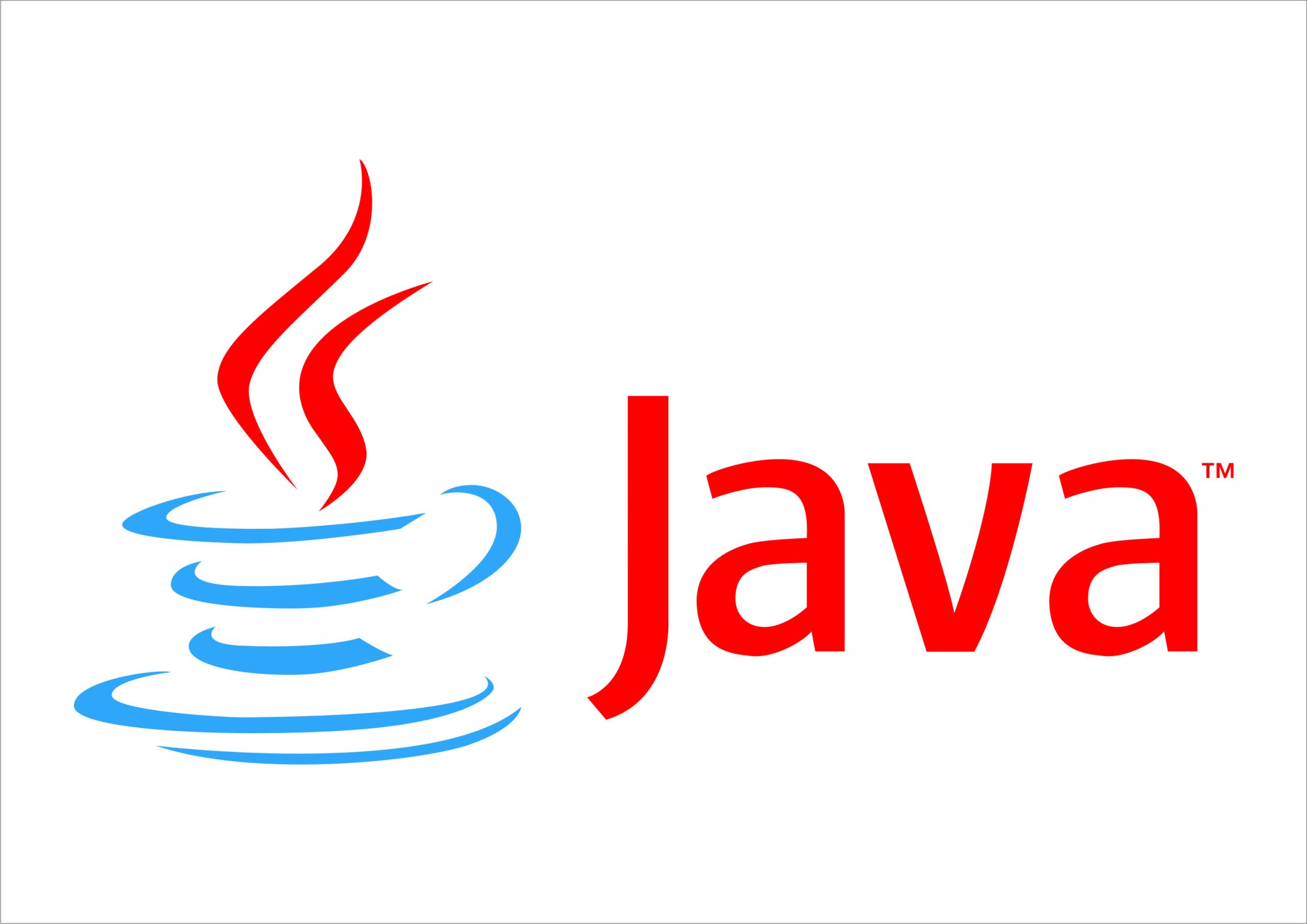
Java使用jdbc连接mysql数据库
连接时需要注意的几点:
1. 将jdbc的jar包放入项目文件lib中
2. 记住mysql安装时的用户名和密码
3. 尽量将类文件放在util目录下
4. 在本地使用时一般只需要改数据库user和pass还有databasename
5. 在其他类中调用完毕后记得关闭连接
6. 新建一个文件名为DBUtil再将代码放入即可
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 |
public class DBUtil { public static String username="root"; public static String password="root"; public static String url="jdbc:mysql://localhost:3306/databasename"; static{ try { Class.forName("com.mysql.jdbc.Driver"); } catch (ClassNotFoundException e) { // TODO Auto-generated catch block e.printStackTrace(); } } //获取到数据库的链接 public static Connection getConnectDb() { Connection conn=null; try { conn = DriverManager.getConnection(url,username,password); } catch (SQLException e) { // TODO Auto-generated catch block e.printStackTrace(); } return conn; } //关闭数据库 public static void CloseDB(ResultSet rs, PreparedStatement stm, Connection conn) { if(rs!=null) { try { rs.close(); } catch (SQLException e) { // TODO Auto-generated catch block e.printStackTrace(); } } if(stm!=null) { try { stm.close(); } catch (SQLException e) { // TODO Auto-generated catch block e.printStackTrace(); } } if(conn!=null) { try { conn.close(); } catch (SQLException e) { // TODO Auto-generated catch block e.printStackTrace(); } } } } |