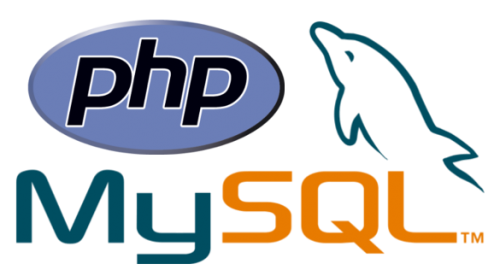
PHP+MySQL项目开发细节总结
- 写出项目流程,要哪些页面,列出提纲
-
创建数据库
-
创建配置文件dbconfig.php
-
创建页面,添加信息
-
在需要的页面执行相应的操作处理,主要是添加,删除,修改等信息操作处理
操作数据库时
-
先导入数据库配置文件
-
获取要执行的信息
-
拼接SQL语句,并执行操作。一般是用switch语句实现,根据传递过来的变量做出处理。
-
对于一些可能会抛出异常的信息用@来屏蔽
例如:
$sql="select * from news where order by addtime desc";
//将最新的新闻先显示出来
$result=@mysql_query($sql,$conn);
在php+mysql开发有关数据库的项目常用的语句:
- 配置文件中的连接mysql服务器,选择数据库,设置编码
1 2 3 4 5 6 7 |
<?php header("content-Type:text/html;charset=utf-8"); //连接数据库配置,主机,数据库用户,密码 $conn=@mysql_connect("localhost","root","1234")or die("error:".mysql_error()); mysql_select_db("newsdb",$conn);//数据库名选择 mysql_query("set names utf8");//设置数据库编码 |
- 添加操作
根据上一次插入的数据的id来判断使用函数:mysql_insert_id($conn)>0来做出处理。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
$sql="insert into news values(null,'{$title}','{$keywords}','{$author}','{$addtime}','{$content}')"; //echo $sql; //insert into news values(null,'大大','2','2','1367984131','4') //3 判断是否成功 if(empty($content)){ die("内容不能为空!"."<a href='add.php'>添加新闻信息</a>"); } mysql_query($sql,$conn); if(mysql_insert_id($conn)>0){ echo "添加成功!"; }else{echo "添加失败!".mysql_error();} echo "<a href='javascript:window.history.back();'>返回</a>";//返回 echo "<br><a href='index.php'>浏览新闻信息</a>"; |
- 删除信息
通过影响的行数mysql_affected_rows($conn)>0;来判断是否删除成功
1 2 3 4 5 6 7 8 |
$sd=$_GET['id']; $sql="delete from news where id={$sd}"; mysql_query($sql,$conn); if(mysql_affected_rows($conn)>0){ echo "删除成功!"; }else{echo "删除失败!".mysql_error();} echo "<br><a href='index.php'>浏览新闻信息</a>"; |
4 更新信息操作
也是用影响的行数来判断
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
$id=$_POST['id']; $title=$_POST['title']; $keywords=$_POST['keywords']; $author=$_POST['author']; $content=$_POST['content']; $sql="update news set title='{$title}',keywords='{$keywords}',author='{$author}',content='{$content}' where id={$id}"; //判断是否打通线 //echo $sql; mysql_query($sql,$conn); if(mysql_affected_rows($conn)>0){ echo "<script>alert('修改成功!');window.location='index.php';</script>"; }else{ echo "<script>alert('修改失败!');history.go(-1);</script>"; } //header("location:index.php"); |
无论是增,删,改,查等操作,重心是理清逻辑思路,然后做出响应的操作。
- switch...case语句在执行信息的增加,删除,修改上发挥了很关键的重要。
比如说:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 |
<?php header("content-Type:text/html;charset=utf-8"); //导入数据库库配置文件 require("dbconfig.php"); switch($_GET['action']){ case "add": //执行添加 //1 获取要添加的信息,并补充其他信息 $title=$_POST['title']; $keywords=$_POST['keywords']; $author=$_POST['author']; $content=$_POST['content']; $addtime=time(); //2 拼接sql语句,并执行添加操作 $sql="insert into news values(null,'{$title}','{$keywords}','{$author}','{$addtime}','{$content}')"; //echo $sql; //insert into news values(null,'大大','2','2','1367984131','4') //3 判断是否成功 if(empty($content)){ die("内容不能为空!"."<a href='add.php'>添加新闻信息</a>"); } mysql_query($sql,$conn); if(mysql_insert_id($conn)>0){ echo "添加成功!"; }else{echo "添加失败!".mysql_error();} echo "<a href='javascript:window.history.back();'>返回</a>";//返回 echo "<br><a href='index.php'>浏览新闻信息</a>"; break; case "del": $sd=$_GET['id']; $sql="delete from news where id={$sd}"; mysql_query($sql,$conn); if(mysql_affected_rows($conn)>0){ echo "删除成功!"; }else{echo "删除失败!".mysql_error();} echo "<br><a href='index.php'>浏览新闻信息</a>"; break; case "update": //获取要修改的信息 $id=$_POST['id']; $title=$_POST['title']; $keywords=$_POST['keywords']; $author=$_POST['author']; $content=$_POST['content']; $sql="update news set title='{$title}',keywords='{$keywords}',author='{$author}',content='{$content}' where id={$id}"; //判断是否打通线 //echo $sql; mysql_query($sql,$conn); if(mysql_affected_rows($conn)>0){ echo "<script>alert('修改成功!');window.location='index.php';</script>"; }else{ echo "<script>alert('修改失败!');history.go(-1);</script>"; } //header("location:index.php"); break; } ?> |