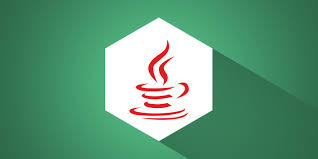
Java网络编程
Java网络API
1. InetAddress类
方法 | 功能 |
---|---|
public static InetAddress getLocalHost | 获取本机对应的InetAddress对象 |
public static InetAddress getByName(String host) | 根据主机获得对应的InetAddress对象,参数host可以是ip地址或域名 |
public static InetAddress[] getAllByName(String host) | 根据主机获得具有相同名字的一组InetAddress对象 |
public static InetAddress getByAddress(byte[] addr) | 获取a对象ddr所封装的IP地址对应的InetAddress |
public String getCanonicalHost() | 获取此IP地址的全限定域名 |
public bytes[] getHostAddress() | 获取该InetAddress对象对应的IP地址字符串 |
public String getHostName() | 获取该InetAddress对象的主机名称 |
public boolean isReachable(int timeout) | 判断是否可以到达该地址 |
示例代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 |
package com.rain.demo; import java.io.IOException; import java.net.InetAddress; import java.net.UnknownHostException; /** * Created by w-pc on 2017/02/27. * java网络编程 */ public class InetAddressDemo { public static void main(String[] args) { try { //本机操作,获取本机地址信息 InetAddress loca = InetAddress.getLocalHost(); System.out.println("本机的全限定域名:"+loca.getCanonicalHostName()); System.out.println("本机的IP地址:"+loca.getHostAddress()); System.out.println("本机的主机名称:"+loca.getHostName()); System.out.println("本机的名称与IP:"+loca.toString()); System.out.println("是否可以到达:"+loca.isReachable(5000)); System.out.println("*********************************************************"); //访问我服务器上的博客 InetAddress loca_blog = InetAddress.getByName("www.rainweb.site"); System.out.println("博客的全限定域名:"+loca_blog.getCanonicalHostName()); System.out.println("博客的IP地址:"+loca_blog.getHostAddress()); System.out.println("博客的主机名称:"+loca_blog.getHostName()); System.out.println("博客的名称与IP:"+loca_blog.toString()); System.out.println("是否可以到达:"+loca_blog.isReachable(5000)); System.out.println("*********************************************************"); //访问测试IP地址127.0.0.1 InetAddress loca_try = InetAddress.getByAddress(new byte[]{127,0,0,1}); System.out.println("全限定域名:"+loca_try.getCanonicalHostName()); System.out.println("IP地址:"+loca_try.getHostAddress()); System.out.println("主机名称:"+loca_try.getHostName()); System.out.println("名称与IP:"+loca_try.toString()); System.out.println("是否可以到达:"+loca_try.isReachable(5000)); } catch (UnknownHostException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); } } } |
结果
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
本机的全限定域名:W-PC 本机的IP地址:192.168.225.1 本机的主机名称:W-PC 本机的名称与IP:W-PC/192.168.225.1 是否可以到达:true ********************************************************* 博客的全限定域名:59.111.97.112 博客的IP地址:59.111.97.112 博客的主机名称:www.rainweb.site 博客的名称与IP:www.rainweb.site/59.111.97.112 是否可以到达:true ********************************************************* 全限定域名:127.0.0.1 IP地址:127.0.0.1 主机名称:127.0.0.1 名称与IP:127.0.0.1/127.0.0.1 是否可以到达:true |
2. URL类
protocol://host:port/resourceName
- protocol是协议名
- host是主机名
- port是端口号
- resourceName是资源名
方法 | 功能描述 |
---|---|
public URL(String spec) | 构造方法,根据指定的字符串来创建一个URL对象 |
public URL(String protocol,String host,int port,String file) | 构造方法,根据指定的协议,主机名,端口号和文件资源来创建一个URL对象 |
public URL(String protocol String host,String file) | 构造方法,根据指定的协议,主机名和文件资源来创建URL对象 |
方法 | 功能描述 |
---|---|
public String getProtocol | 返回协议名 |
public String getHost() | 返回主机名 |
public int getPort() | 返回端口号,如没有则返回-1 |
public String getFile() | 返回文件名 |
public String getRef() | 返回URL的锚 |
public String getQuery() | 返回URL的查询信息 |
public String getPath() | 返回URL的路径 |
public URLConnection openConnection() | 返回一个URLConnection对象 |
public final InputStream openStream() | 返回一个用于读取该URL资源的InputStream流 |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 |
package com.rain.demo; import java.net.MalformedURLException; import java.net.URL; /** * Created by w-pc on 2017/03/08. */ public class URLDemo { public static void main(String[] args) { String url = "http://www.rainweb.site:80/index.php"; try { URL blog = new URL(url); System.out.println("返回协议名:"+blog.getProtocol()); System.out.println("返回主机名:"+blog.getHost()); System.out.println("返回端口号:"+blog.getPort()); System.out.println("返回文件名:"+blog.getFile()); System.out.println("返回URL的锚:"+blog.getRef()); System.out.println("返回URL的查询信息:"+blog.getQuery()); System.out.println("返回URL的路径:"+blog.getPath()); System.out.println(""); }catch (MalformedURLException e){ e.printStackTrace(); } } } |
结果:
1 2 3 4 5 6 7 8 |
返回协议名:http 返回主机名:www.rainweb.site 返回端口号:80 返回文件名:/index.php 返回URL的锚:null 返回URL的查询信息:null 返回URL的路径:/index.php |
3.URLConnection类
URLConnection代表与URL指定的数据源的动态连接,该类提供一些比URL类更强大的服务器交互控制的方法,允许使用POST或PUT和其他HTTP请求方法将数据送回服务器。URLConnection是一个抽象类。
方法 | 功能描述 |
---|---|
public int getContentLength() | 获得文件的长度 |
public String getContentType() | 获得文件的类型 |
public long getDate() | 获得文件创建的时间 |
public long getLastModified() | 获得文件最后修改的时间 |
public InputStream getInputStream() | 获得输入流,以便读取文件的数据 |
public OuputStream getOutputStream() | 获得输出流,以便输出数据 |
public void setRequestProperty(String key,String value) | 设置请求属性值 |
获取网页源代码
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 |
package com.rain.demo; import java.io.BufferedReader; import java.io.IOException; import java.io.InputStreamReader; import java.net.MalformedURLException; import java.net.URL; import java.net.URLConnection; /** * Created by w-pc on 2017/03/08. */ public class URLDemo { public static void main(String[] args) { String url = "http://www.rainweb.site:80/index.php"; try { //构建URL对象 URL blog = new URL(url); //由URL对象获取URLConnection对象 URLConnection urlConn = blog.openConnection(); //设置请求属性,字符集 urlConn.setRequestProperty("Charset", "UTF-8"); //由URLConnection获取输入流,并构造BufferedReader对象 BufferedReader br = new BufferedReader(new InputStreamReader(urlConn.getInputStream())); String inputLine; while ((inputLine = br.readLine()) != null){ System.out.println(inputLine); } br.close(); }catch (MalformedURLException e){ e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); } } } |
4.URKDecoder类和URLEncoder类的使用
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
package com.qst.chapter06; import java.io.UnsupportedEncodingException; import java.net.URLDecoder; import java.net.URLEncoder; public class URLDecoderDemo { public static void main(String[] args) { try { // 将普通字符串转换成application/x-www-form-urlencoded字符串 String urlStr = URLEncoder.encode("Java 8高级应用与开发", "GBK"); System.out.println(urlStr); // 将application/x-www-form-urlencoded字符串 转换成普通字符串 String keyWord = URLDecoder.decode( "Java+8%B8%DF%BC%B6%D3%A6%D3%C3%D3%EB%BF%AA%B7%A2", "GBK"); System.out.println(keyWord); } catch (UnsupportedEncodingException e) { e.printStackTrace(); } } } |